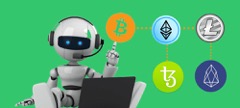
Cryptocurrency Trading Bots
-
7 mins
Cryptocurrency trading has boomed in recent years. High volatility and trading volume in cryptocurrencies suit short-term trading very well.
I think Dollar Cost Averaging (DCA) is the right trading technique for Cryptocurrency. DCA means investing a set amount of money into an asset regularly, disregarding the price action.
With the rise of Automation and DCA Bots, traders should get started with DCA bots. With DCA, one can divide the total investment into smaller pieces and buy the asset at various points over time at different prices, thereby getting a better average price for the position and greatly reducing risks from the consequences of volatility.
Here I provide sample python code for DCA Cryptocurrency trading Bots using with 3Commas APIs.
Requirements
Python Installation
File: requirements.txt
py3cw==0.0.31
PyYAML==6.0
times==0.7
Sample Code
File: 3commas_bot.py
from py3cw.request import Py3CW
import json
import yaml
import sys
import os
import time
secret_file = 'src/3cw_secret_key.conf'
def get_secret_key(secret_file):
p3cw = Py3CW(key="unknown", secret="unknown")
with open(secret_file, 'r') as yaml_in:
parsed_yaml = yaml.load(yaml_in, Loader=yaml.FullLoader)
my_key = parsed_yaml["key"]
my_secret = parsed_yaml["secret"]
p3cw = Py3CW(key=my_key,secret=my_secret)
return p3cw
File: 3commas_bot.py
def connected_accounts(p3cw):
error, data = p3cw.request(
entity='accounts',
action= ''
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def load_balance(p3cw, account_id):
error, data = p3cw.request(
entity='accounts',
action='load_balances',
action_id=str(account_id),
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def get_bots(p3cw):
error, data = p3cw.request(
entity='bots',
action= ''
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def enable_bot_id(p3cw, id):
error, data = p3cw.request(
entity='bots',
action= 'enable',
action_id=str(id),
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def get_deals_stats_bot_id(p3cw, id):
error, data = p3cw.request(
entity='bots',
action= 'deals_stats',
action_id=str(id),
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def cancel_all_deals_bot_id(p3cw, id):
error, data = p3cw.request(
entity='bots',
action= 'cancel_all_deals',
action_id=str(id),
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def start_new_deal_bot_id(p3cw, id):
error, data = p3cw.request(
entity='bots',
action= 'start_new_deal',
action_id=str(id),
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def disable_bot_id(p3cw, id):
error, data = p3cw.request(
entity='bots',
action= 'disable',
action_id=str(id),
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def get_bot_id(p3cw, id):
error, data = p3cw.request(
entity='bots',
action= 'show',
action_id=str(id),
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def update_bot_id(p3cw, id, bot_params):
error, data = p3cw.request(
entity='bots',
action= 'update',
action_id=str(id),
payload=bot_params,
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
def create_bot(p3cw, bot_params):
error, data = p3cw.request(
entity='bots',
action= 'create_bot',
payload=bot_params,
)
if error == {}:
return data
else:
raise(Exception(error['msg']))
File: BOT_BTC_USD.yaml
Here is a sample strategy for trading BTC. It has deal start conditions based on TradingView signals.
3commas trading bot config
#Main settings
account_id: 12345678
account_name: Binance US
name: BTC_USD
id: 9876511
is_enabled: false
type: Bot::SingleBot
#Pairs
pairs:
- USD_BTC
#Strategy
strategy: long
start_order_type: limit
profit_currency: base_currency
base_order_volume: '50.0'
safety_order_volume: '60.0'
base_order_volume_type: quote_currency
safety_order_volume_type: quote_currency
profit_currency: quote_currency
#Deal start condition
strategy_list:
- options:
time: 1m
type: buy_or_strong_buy
strategy: trading_view
- options:
time: 5m
type: buy_or_strong_buy
strategy: trading_view
- options:
time: 15m
type: buy_or_strong_buy
strategy: trading_view
- options:
points: '50'
time: 3m
strategy: rsi
#Take profit
take_profit: '2.00'
take_profit_type: total
trailing_deviation: '0.2'
trailing_enabled: true
#Safety orders
max_safety_orders: 18
active_safety_orders_count: 1
safety_order_step_percentage: '3.0'
martingale_step_coefficient: '1.0'
martingale_volume_coefficient: '1.05'
max_active_deals: 2
max_price: null
Running Bot
>dir bot_config
BOT_BTC_USD.yaml
>python3 3commas_bot.py bot_config/
Conclusion
Automated Trading Bots have many advantages like Minimizing Emotions, Backtesting, & Preserving Discipline . Although Automated Trading is appealing, it should not be considered a substitute for carefully executed trading. Automated Trading does require constant monitoring and fine-tuning to get the desired results. Remember to leverage trading platforms such as 3Commas to get some trading experience and knowledge to jump-start using automated trading systems.
DISCLAIMER: All views expressed on this site are my own and do not represent the opinions of any entity whatsoever with which I have been, am now, or will be affiliated. Any collateral used is referenced in the Web Resources or others sections on this page. The information provided on this website does not constitute investment advice, financial advice, or trading advice.